.DS_Store
# Learn ES6 (ECMAScript 2015)
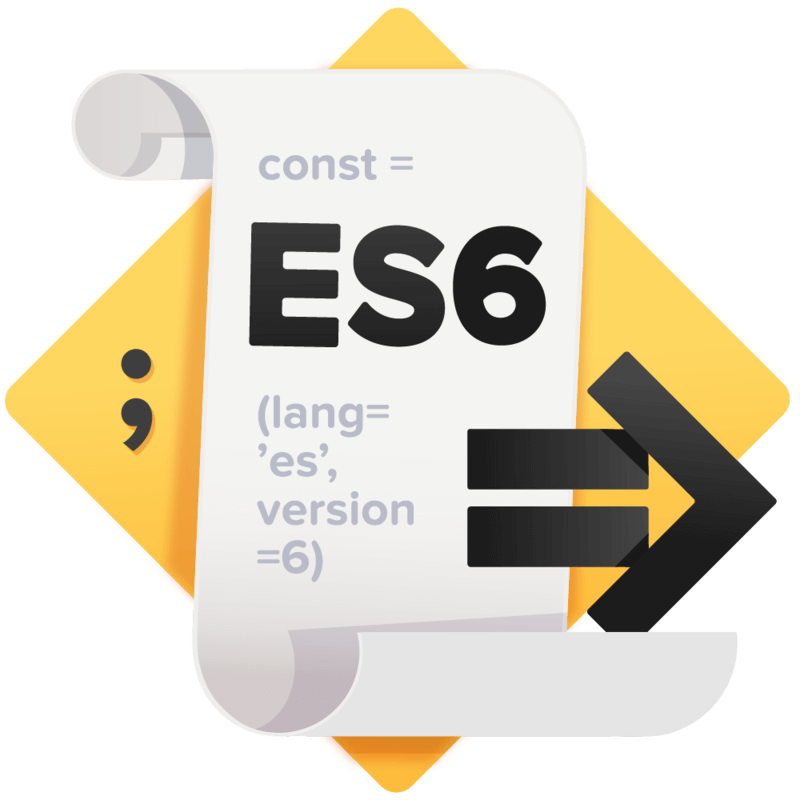
This course takes a look at some of the new features that JavaScript has available with ES6 (ECMAScript 2015). It is a "montage" from several instructors.
Each lesson's code is in its corresponding lesson folder. Plunks are drawn from the lesson's branch.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Plunker</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
//rest parameters vs arguments keyword
function Store() {
var aisle = {
fruit: [],
vegetalbe: []
}
return {
//Store().add('category', 'item1', 'item2');
add: function(category, ...items) {
//var items = [].splice.call(arguments, 1);
console.log(items) || displayInPreview(items);
items.forEach(function(value, index, array) {
aisle[category].push(value);
});
},
aisle: aisle
}
}
var myGroceryStore = new Store();
myGroceryStore.add('fruit', 'apples', 'oranges');
console.log(myGroceryStore.aisle) || displayInPreview(myGroceryStore.aisle.fruit);
// display in plunker preview
function displayInPreview(string) {
var newDiv = document.createElement("div");
var newContent = document.createTextNode(string);
newDiv.appendChild(newContent);
document.body.appendChild(newDiv)
}