.DS_Store
# Learn ES6 (ECMAScript 2015)
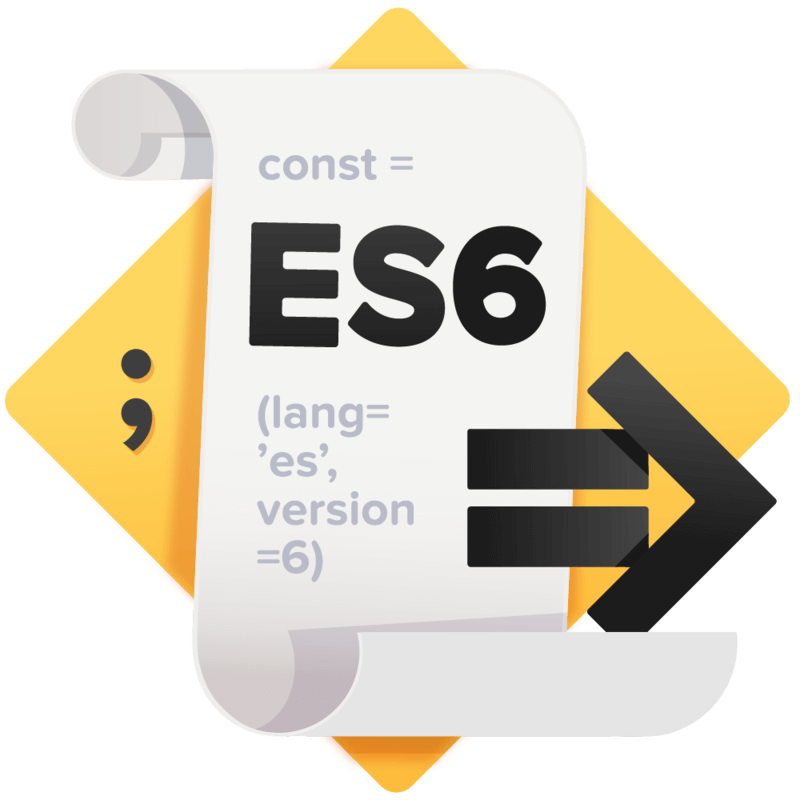
This course takes a look at some of the new features that JavaScript has available with ES6 (ECMAScript 2015). It is a "montage" from several instructors.
Each lesson's code is in its corresponding lesson folder. Plunks are drawn from the lesson's branch.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Plunker</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
var people = [
{
"firstName": "Clinton",
"lastName": "Ruiz",
"phone": "1-403-985-0449",
"email": "pharetra@facilisislorem.org",
"address": "Ap #829-3443 Nec St."
},
{
"firstName": "Skyler",
"lastName": "Carroll",
"phone": "1-429-754-5027",
"email": "Cras.vehicula.alique@diamProin.ca",
"address": "P.O. Box 171, 1135 Feugiat St."
},
{
"firstName": "Kylynn",
"lastName": "Madden",
"phone": "1-637-627-2810",
"email": "mollis.Duis@ante.co.uk",
"address": "993-6353 Aliquet, Street"
},
{
"firstName": "Chaney",
"lastName": "Edwards",
"phone": "1-397-181-4501",
"email": "rutrum@Nullamlobortis.net",
"address": "P.O. Box 342, 9574 Egestas Street"
}
]
people.forEach(({firstName}) => console.log(firstName) || displayInPreview(firstName))
var [,Skyler] = people;
function logEmail({email}){
console.log(email) || displayInPreview(email);
}
logEmail(Skyler);
// display in plunker preview
function displayInPreview(string) {
var newDiv = document.createElement("div");
var newContent = document.createTextNode(string);
newDiv.appendChild(newContent);
document.body.appendChild(newDiv)
}