<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="initial-scale=1, maximum-scale=1, user-scalable=no, width=device-width" />
<title>Franken Talks</title>
<link data-require="ionic@1.0.0-beta13" data-semver="1.0.0-beta13" rel="stylesheet" href="http://code.ionicframework.com/1.0.0-beta.13/css/ionic.css" />
<link href="css/extra.css" rel="stylesheet" />
<script data-require="ionic@1.0.0-beta13" data-semver="1.0.0-beta13" src="http://code.ionicframework.com/1.0.0-beta.13/js/ionic.bundle.js"></script>
<script src="js/app.js"></script>
<script src="js/talks.js"></script>
</head>
<body ng-app="app" animation="slide-left-right-ios7">
<div>
<div>
<ion-nav-bar class="bar-stable">
<ion-nav-back-button class="button-icon icon ion-ios7-arrow-back">Back</ion-nav-back-button>
</ion-nav-bar>
<ion-nav-view></ion-nav-view>
</div>
</div>
</body>
</html>
This demo app will be created during my conference talk at the MDC kompakt 2014. The slides are available at [http://johanneshoppe.github.io/IonicPresentation/](http://johanneshoppe.github.io/IonicPresentation/)
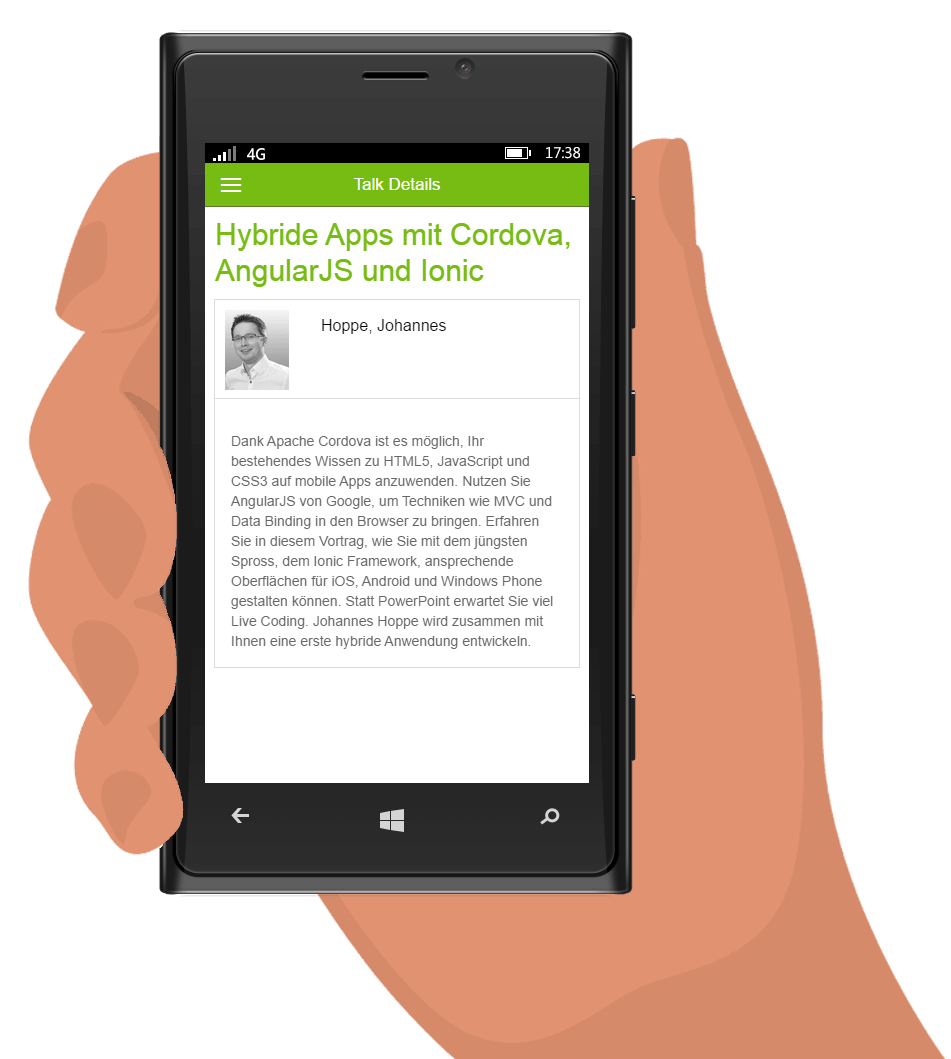
.bar.bar-positive {
background-color: #76bc13;
border-color: #5b7930;
}
h1 {
color: #76bc13;
}
.scroll-content h1 {
font-size: 30px;
}
angular.module('app', ['ionic'])
.config(function ($stateProvider, $urlRouterProvider) {
$stateProvider
.state('side-menu1', {
url: '/menu',
templateUrl: 'side-menu1.html',
controller: function ($scope, $ionicSideMenuDelegate, $timeout, talksService) {
$scope.talks = [
{
"title": "Lade Daten",
"description": "Bitte warten Sie einen Moment, bis die Datan geladen sind.",
"start": null,
"speaker": null
}
];
talksService.loadTalkData().then(function(talks) {
$scope.talks = talks;
$scope.activeTalk = $scope.talks[talksService.getLastActiveIndex()];
});
$scope.selectTalk = function (talk, index) {
$scope.activeTalk = talk;
talksService.setLastActiveIndex(index);
$ionicSideMenuDelegate.toggleLeft(false);
};
$timeout(function () {
$ionicSideMenuDelegate.toggleLeft(true);
});
}
});
$urlRouterProvider.otherwise('/menu');
});
angular.module('app')
/**
* The Talks factory handles saving and loading talks
* from local storage, and also lets us save and load the
* last active talk index.
*/
.factory('talksService', function($window, $http, $q) {
var url = 'http://johanneshoppe.github.io/IonicPresentation/Demos/2015_Franken_Demo/talks_callback.json';
// tries to load talk data from internet, or uses localStorage as fallback
var loadTalkData = function() {
var deferred = $q.defer();
$http.jsonp(url)
.success(function(talks) {
window.localStorage.setItem('talks', angular.toJson(talks));
deferred.resolve(talks);
})
.error(function() {
var talksString = $window.localStorage.getItem('talks');
var talks = talksString ? angular.fromJson(talksString) : [];
deferred.resolve(talks);
});
return deferred.promise;
};
var getLastActiveIndex = function() {
return parseInt($window.localStorage.getItem('lastActiveTalk'), 10) || 0;
};
var setLastActiveIndex = function(index) {
$window.localStorage.setItem('lastActiveTalk', index + "");
}
return {
loadTalkData: loadTalkData,
getLastActiveIndex: getLastActiveIndex,
setLastActiveIndex: setLastActiveIndex
}
})
.filter('imagePath', function() {
return function (talk) {
if (!talk) {
return undefined;
}
var r = talk.speaker.toLowerCase();
r = r.replace(new RegExp("\\s", 'g'), "_");
r = r.replace(new RegExp("[àáâãäå]", 'g'), "a");
r = r.replace(new RegExp("æ", 'g'), "ae");
r = r.replace(new RegExp("ç", 'g'), "c");
r = r.replace(new RegExp("[èéêë]", 'g'), "e");
r = r.replace(new RegExp("[ìíîï]", 'g'), "i");
r = r.replace(new RegExp("ñ", 'g'), "n");
r = r.replace(new RegExp("[òóôõö]", 'g'), "o");
r = r.replace(new RegExp("œ", 'g'), "oe");
r = r.replace(new RegExp("[ùúûü]", 'g'), "u");
r = r.replace(new RegExp("[ýÿ]", 'g'), "y");
r = r.replace("&", "_");
r = r.replace(",", "_");
return "http://johanneshoppe.github.io/IonicPresentation/Demos/2015_Franken_Demo/FrankenTalks/img/" + r + ".png";
};
});
<ion-side-menus>
<!-- Left menu -->
<ion-side-menu side="left">
<ion-header-bar class="bar-positive">
<img src="http://johanneshoppe.github.io/IonicPresentation/Demos/2015_Franken_Demo/FrankenTalks/img/logo-franken.png" width="50" height="50" /><h1 class="title">Talks</h1>
</ion-header-bar>
<ion-content padding="false" class="has-header">
<ion-list>
<ion-item
ng-repeat="talk in talks"
ng-click="selectTalk(talk, $index)" ng-class="{ active: activeTalk == talk }">
<b ng-bind="talk.start | date:'HH:mm'" class="item-note"></b>
<span ng-bind="talk.title">Talk Titel</span>
</ion-item>
</ion-list>
</ion-content>
</ion-side-menu>
<!-- Center content -->
<ion-pane ion-side-menu-content>
<ion-header-bar class="bar-positive">
<button class="button button-icon" menu-toggle="left">
<i class="icon ion-navicon"></i>
</button>
<h1 class="title">Talk Details</h1>
</ion-header-bar>
<ion-content padding="true" class="has-header">
<h1 ng-bind="activeTalk.title">Talk Title</h1>
<ion-list>
<ion-item class="item-thumbnail-left">
<img src="img/avatar-img.png" ng-src="{{activeTalk | imagePath}} " style="width: auto;">
<h2 ng-bind="activeTalk.speaker">Name</h2>
<p ng-bind="activeTalk.start | date: "dd.MM.yyyy - HH:mm 'Uhr'"">Uhrzeit</p>
</ion-item>
<ion-item class="item-body">
<p ng-bind="activeTalk.description">Beschreibung</p>
</ion-item>
</ion-list>
</ion-content>
</ion-pane>
</ion-side-menus>